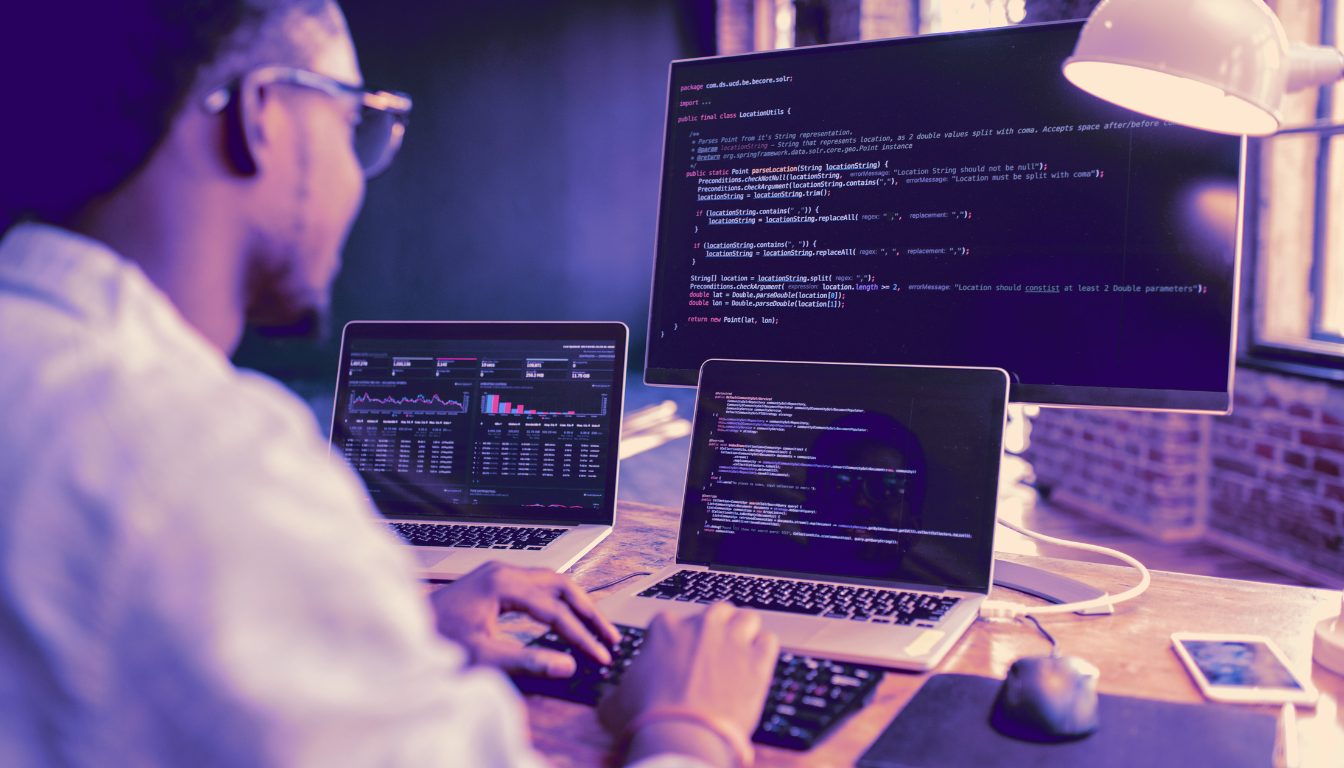
The Critical Role of Object Classes in Programming, and How They Work
Post Author:
CacheFly Team
Categories:
Date Posted:
January 20, 2024
Key Takeaways
- Understanding the concept of object classes in programming and their role in object-oriented programming.
- Recognizing the importance of object class grouping in managing objects in a program.
- Identifying examples of object classes in real-world scenarios like e-mail systems.
- Grasping the intricacies of how classes serve as blueprints for objects, defining the properties and methods that an object can possess.
As we delve into object-oriented programming, we must grasp the core concept that drives it – object classes. These classes are central in structuring code and creating instances, from defining properties to bundling methods. Moreover, grouping object classes is a linchpin in managing objects in a program, especially when handling large systems. But how does this work in real-world scenarios? Let’s explore how something as ubiquitous as an e-mail system can serve as an example of an object class.
Defining Object Classes in Programming
At the heart of object-oriented programming lies the concept of object classes. These classes function as a blueprint for objects, outlining the properties and methods that an object can possess. Look at it as the DNA of an object, carrying the information that determines its characteristics and behavior. This concept is the cornerstone of structuring code and creating instances in object-oriented programming.
So, what role do classes play in object-oriented programming? The answer lies in the way they allow for grouping. Object classes group objects that share common attributes, a feature that becomes indispensable when managing objects in a program, especially when dealing with large systems. It’s like having a well-organized toolbox where every tool has its designated place, making it easier to find and use when needed.
But how does this play out in real-world scenarios? Let’s take a look at e-mail systems. Believe it or not, an e-mail system could be an example of an object class. How so? All e-mail systems have certain things in common — sending, receiving, storing, and displaying messages. These shared attributes can be defined in a class, and each e-mail system can be an instance of that class, each with unique properties like user interface design or spam filtering algorithm. This demonstrates how object classes can be applied to practical, everyday systems we interact with.
Understanding the concept of object classes and their application in programming is the first step toward mastering object-oriented programming. So, remember these points as you navigate the fascinating world of object classes!
Working with Objects and Instances in Programming
In the programming world, ‘object’ is a term you’ll often hear tossed around. But what does it mean? In simple terms, an object refers to an instance of a class. This might sound a little abstract, but think of it this way: If a class is a blueprint, an object is the building constructed using that blueprint. Each building constructed using the same blueprint shares some characteristics but can also have its unique features. Similarly, each class object shares some properties and methods defined in the class but can also have its unique properties.
Creating Objects in JavaScript
JavaScript is a great language to illustrate how objects are created and used. In JavaScript, creating an object is as simple as defining variable values. You can access and modify the object’s properties using dot or bracket notation. This makes interacting with objects in JavaScript incredibly intuitive and flexible.
But remember, JavaScript is a prototype-based language, meaning objects inherit directly from other objects. This is a different concept from class-based inheritance found in languages like Java, where inheritance is defined between classes, and objects are instances of these classes.
ObjectClass vs Object: What’s the Difference?
As you delve deeper into programming, you’ll encounter terms like ObjectClass and Object. While they sound similar, they refer to different things. ObjectClass typically refers to a class, the blueprint that defines what an object should look like and how it should behave. On the other hand, an Object relates to an instance of a class — a tangible manifestation of the blueprint. Understanding this distinction is crucial in grasping object-oriented programming concepts.
Determining the Class of an Object in Java
In some programming scenarios, you may need to determine the class of an object. Java provides various ways to do this, each applicable in different contexts. For example, the getClass() method can be used to fetch the runtime class of an object. The instanceof operator, on the other hand, checks whether an object is an instance of a certain class. And the isInstance() method works similarly to the instanceof operator but is less commonly used. This Java program offers a practical example of determining an object’s class.
Working with objects and instances is a core aspect of programming. Mastering this concept is a significant stride towards proficiency in object-oriented programming. So, as you continue your journey, remember to use these concepts and techniques to your advantage.
Understanding the Role of Object Storage in Programming
As we delve deeper into object-oriented programming, we encounter a crucial concept: object storage. Object storage is a data storage architecture that treats data as objects, contrary to other storage architectures like file systems or block storage that manage data as a file hierarchy or as fixed-sized blocks within sectors respectively. This shift from traditional file or block storage to object storage has significant implications for managing and accessing data in programming.
The Benefits of Object Storage
One of the standout benefits of object storage is its ability to handle “read many, write few” requests efficiently. This trait makes object storage particularly effective in machine and deep learning models, where large volumes of data need to be read multiple times but rarely modified. In addition, object storage scales horizontally, meaning you can add more storage nodes to the system to increase capacity, which is a significant advantage in today’s data-driven world.
Object Storage and CDN: A Powerful Combination
Object storage can significantly enhance the performance of a Content Delivery Network (CDN). How does this work? CDNs distribute content to end-users with high availability and performance, and object storage can store this content efficiently and flexibly. By integrating object storage with a CDN, you can optimize content delivery and performance, ensuring that your end-users always get the best experience.
Use Cases of Object Storage in Programming
Object storage has a wide range of applications in programming. As mentioned earlier, one notable use case is in machine and deep learning models. These models require access to large amounts of data, which object storage can handle efficiently. But that’s not all. Object storage is also beneficial in web applications, storing user-generated content like photos and videos. It can also be used in big data analytics to store large volumes of unstructured data.
Understanding object storage and its benefits can open up new possibilities in your programming projects. You can enhance data management and performance by leveraging object storage, creating more efficient and robust applications.
Reassessing the Role of Classes in JavaScript
While we commonly associate object classes with the building blocks of JavaScript code, we mustn’t confine ourselves to this perspective. Despite their prevalence, classes in JavaScript shouldn’t necessarily be the preferred method of organizing code. Recognizing this helps us understand the versatility of JavaScript and appreciate the variety of techniques we can employ.
Unpacking the Predicament with Classes in JavaScript
Classes can sometimes complicate the coding process in JavaScript. JavaScript is a prototype-based language, and while classes provide a more straightforward and familiar programming model, they can sometimes obscure the language’s true nature. Understanding what classes are and how they function can aid in making informed decisions about whether to use them in your coding projects.
Diversifying Your Coding Techniques: Alternatives to Object Classes
Fortunately, JavaScript offers a range of alternatives to using classes, some of which may prove more efficient and effective depending on your specific project needs. These alternatives can introduce greater flexibility and simplicity into your code, helping you to write more readable and maintainable programs.
Exploring Examples of Alternatives to Classes in JavaScript
Let’s delve into some alternatives to using object classes in JavaScript. One such alternative is functional programming, a paradigm that treats computation as evaluating mathematical functions and avoids changing-state and mutable data. Prototypes are another powerful alternative, allowing you to create objects without defining a class. And then there are closures, functions that have access to the parent scope, even after the parent function has closed. Each alternative presents a unique approach to organizing and structuring your code, offering a different perspective to the conventional class-based model.
By exploring these alternatives, you can broaden your understanding of JavaScript and make more informed decisions about how to structure your code. The choice between classes or alternatives depends on the specific needs of your project, and understanding these options can help you choose the most effective approach.
Implementing Object Classes in Practical Programming Scenarios
The theoretical concepts surrounding object classes are essential to grasp, but their practical applications in real-world programming scenarios truly bring these principles to life. Object classes’ inherent ability to structure code, manage complexity, and promote code reuse is integral to various facets of software development.
Practical Applications of Object Classes in Programming
Object classes find usage in a broad spectrum of programming scenarios. Their influence is wide and profound, from creating user interfaces to managing databases. For instance, consider the creation of Graphical User Interface (GUI) components. Object classes are the foundation for creating these components, streamlining the process, and ensuring consistency across the board. Similarly, object classes come into play when managing user sessions in web applications, offering a systematic approach to tracking and maintaining user activity.
The Role of Object Classes in Software Development
Object classes are more than just a theoretical concept in the grand scheme of software development. They are practical tools that developers use to structure their code. They help manage complexity by allowing developers to group related functions and data, creating a blueprint that can be used to create multiple similar objects. Additionally, object classes promote code reuse, a fundamental principle in efficient software development, by enabling developers to create instances of a class whenever needed.
Real-World Examples of Object Class Utilization
In real-world programming scenarios, object classes are pivotal in modeling real-world objects in simulation software. Consider a simulation software for a traffic system. Object classes could represent different entities, such as cars, traffic signals, and pedestrians, with each class encapsulating the relevant properties and behaviors of the respective entity. Such applications of object classes not only make programming concepts relatable but underscore their relevance in practical scenarios.
Future Trends in Object-Oriented Programming
As the programming landscape evolves, so does how we utilize object classes. The rise of new programming paradigms like functional and reactive programming is reshaping how we approach object-oriented programming. These paradigms encourage developers to think beyond the traditional constructs of classes and objects, opening up new possibilities for code structure and organization. While object classes will continue to be a staple in programming, these emerging trends highlight the dynamic and ever-changing nature of the programming world.
Product Updates
Explore our latest updates and enhancements for an unmatched CDN experience.
Book a Demo
Discover the CacheFly difference in a brief discussion, getting answers quickly, while also reviewing customization needs and special service requests.
Free Developer Account
Unlock CacheFly’s unparalleled performance, security, and scalability by signing up for a free all-access developer account today.
CacheFly in the News
Learn About
Work at CacheFly
We’re positioned to scale and want to work with people who are excited about making the internet run faster and reach farther. Ready for your next big adventure?