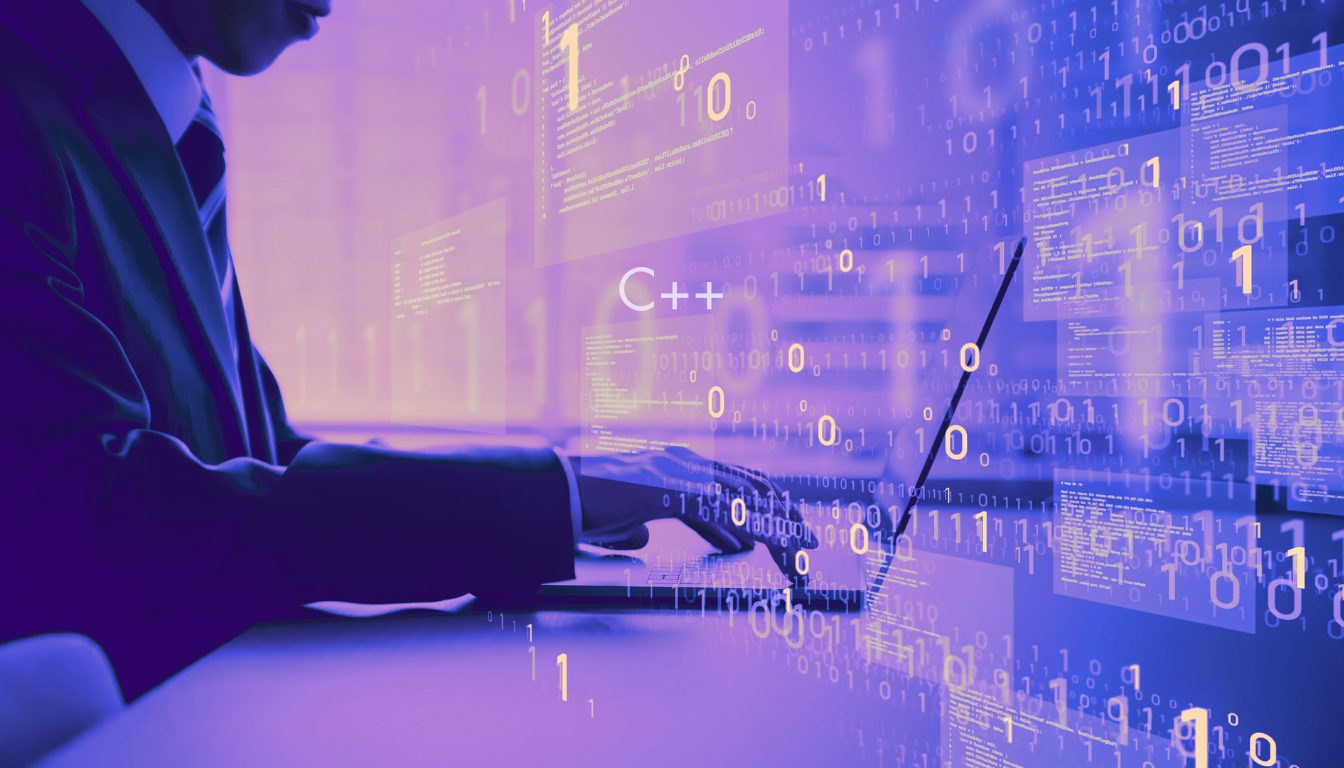
How to Master the Standard Template Library (STL) Effectively
Key Takeaways
- The Standard Template Library (STL) is a software library within the C++ Standard Library designed to provide a collection of generic algorithms, containers, and other components.
- Alexander Stepanov and Meng Lee initially designed the STL, and its design has greatly influenced many parts of the C++ Standard Library.
- One of the main purposes of the STL is to promote reusability across different projects, thus standardizing processes and bringing consistency within an organization.
- Understanding and mastering the STL can help in efficient and optimized content delivery, especially for technical CDN buyers in engineering teams with an advanced technical understanding.
As technological advancements continue to shape our world, the need for efficient systems and tools in software development becomes increasingly important. One such tool that has proven invaluable in programming is the Standard Template Library (STL). This software library, a key part of the C++ Standard Library, was designed to provide a wide range of generic algorithms, containers, and other components that can be used across different projects. The beauty of the STL lies in its ability to standardize processes and bring consistency within an organization. Let’s delve into the origin and development of STL and how it continues to revolutionize content delivery.
Introduction to the Standard Template Library (STL)
The Standard Template Library (STL) is a software library that forms a crucial part of the C++ Standard Library. It was initially designed by Alexander Stepanov and Meng Lee, two renowned computer scientists who contributed significantly to the development of C++. Their vision was to create a library providing a collection of generic algorithms, containers, and other components that could be easily reused across different projects.
This idea of reusability is central to the concept of STL. STL encourages developers to reuse code rather than write new code from scratch for each project by providing ready-to-use components. This saves time and effort, helps standardize processes, and brings consistency within an organization. This is particularly important in large projects where multiple developers work on different system parts.
STL offers various algorithms and data structures commonly used in software development. These include sorting and searching algorithms, multiple types of containers like vectors, lists, and queues, as well as other valuable components like iterators and allocators. By providing these components as part of the library, STL allows developers to focus on the unique aspects of their projects rather than spending time on implementing standard algorithms and data structures.
However, mastering the use of STL requires a good understanding of its structure and the purpose of its various components. In the coming sections, we will dive deeper into the elements of STL, how they work, and how they can be used effectively to enhance your projects. Stay tuned!
Delving into the Components of the Standard Template Library
At the heart of the Standard Template Library lies its four key components: algorithms, containers, functions, and iterators. Together, these elements form the core infrastructure of STL, each playing a crucial role in its functionality and efficiency.
Algorithms
The first component, algorithms, is the backbone of STL. They are implemented to require a certain level of iterator, which is an object that enables a programmer to traverse through a container, such as an array or a list. These algorithms, which range from sorting to complex mathematical computations, are designed to work seamlessly with STL containers. This close relationship between algorithms and iterators is a fundamental aspect of STL’s design, allowing for greater flexibility and efficiency in software development.
Containers
The second component, containers, are objects that store data. They are the building blocks of STL, providing a way for programmers to manage collections of objects. STL boasts a variety of container types, including sequence containers like vectors, deques, and lists, and associative containers such as sets, multisets, maps, multimaps, hash_sets, hash_maps, hash_multisets, and hash_multimaps. Each container has unique properties and use cases, making STL a versatile tool for various programming tasks.
Functions
The third component, functions, are standalone procedures that perform specific tasks. In the context of STL, functions often serve as building blocks for algorithms. They are generally declared within the scope of a class or an object and can be called upon when necessary. Functions contribute to the flexibility and efficiency of STL by allowing repeated sections of code to be grouped into single, reusable units.
Iterators
Last but not least, we have iterators. They are a vital aspect of STL, enabling programmers to access elements stored in a container, regardless of complexity. Iterators are a bridge between containers and algorithms, ensuring the latter can operate on the former. With different types of iterators available, including input, output, forward, bidirectional, and random access, STL provides a versatile and robust framework for software development.
By understanding these four components, you, as a developer, can leverage the full potential of the Standard Template Library. With its focus on reusability and efficiency, STL is an invaluable tool for any programmer, particularly those in the tech industry seeking to deliver high-quality, consistent, and reliable software solutions.
Decoding the Functionality of STL Algorithms
The Standard Template Library’s (STL) power lies significantly in its algorithms. These are instructions or rules designed to perform specific tasks, such as searching and sorting data within containers. Let’s delve deeper into the role and functionality of STL algorithms.
Role of STL Algorithms
STL algorithms are the workhorses that perform various activities on data stored in STL containers. They are implemented to work in harmony with iterators, which act as a bridge between the data and the algorithm. This means that STL algorithms are designed to operate at a certain level of iterator, which specifies the operations that can be performed on the elements of the container.
Advantages of Random-Access Iterators
Among the types of iterators, random-access iterators stand out for their efficiency. Unlike other types, they provide the ability to access elements at any position within the container directly, allowing STL algorithms to process data more quickly. This distinctive feature makes random-access iterators a powerful tool for enhancing the efficiency of your software solutions.
Predicates in STL
Another compelling aspect of STL algorithms is the concept of predicates. A predicate is a function that returns a boolean value based on certain conditions. In STL, predicates are used extensively in search algorithms to specify the conditions under which an element is considered a match. This adds another layer of versatility to STL algorithms, enabling you to tailor your search criteria to specific needs.
Limitations of STL Algorithms
While STL algorithms are compelling, they are not without their limitations. For instance, search operations can be slower on associative containers than on sequence containers. This is because associative containers, such as sets or maps, are designed for efficient lookups of specific elements rather than iterating over a range of components. However, with a deep understanding of these limitations and carefully selecting the appropriate data structure, you can still achieve optimum performance with STL.
In software development, mastering the functionality of STL algorithms is key to delivering efficient and high-quality solutions. As you navigate the complex landscape of the tech industry, the nuanced understanding of these algorithms can serve as a valuable asset in your toolkit.
Navigating the World of STL Containers
Standard Template Library (STL) containers are a fundamental component of the STL. They provide a standardized way to store data that can be manipulated using STL algorithms. Let’s take a closer look at the different types of STL containers and how they function.
Different Types of STL Containers
STL containers are categorized into three types: sequence containers, associative containers, and container adaptors. Sequence containers, such as vector, deque, and list, store elements in a linear sequence. However, associative containers, store data in a way that supports quick retrieval of specific elements. These include set, multiset, map, and multimap containers. Lastly, container adaptors provide a different interface for sequence containers, allowing you to adapt the container’s behavior to fit particular needs.
Functionality and Use Cases
Each type of STL container serves a unique purpose. Sequence containers are commonly used when you need to access elements by their position in the container. Associative containers are ideal when you quickly search for elements based on a key. Meanwhile, container adaptors like stack, queue, and priority_queue are perfect when you need to impose specific rules on inserting and removing elements.
Concept of Ranges in STL
The concept of ranges is integral to STL. Most STL algorithms operate on ranges. These are defined as a pair of iterators specifying the beginning and end of the sequence of elements. This approach provides a high degree of flexibility. It allows STL algorithms to work with any container that provides access to its elements through iterators.
Potential Issues with STL Containers
While STL containers are robust, they come with potential issues. One such issue is the possibility of an iterator becoming invalid after an element has been deleted from the container. Understanding these nuances is crucial to avoid pitfalls and ensure the robustness of your software solutions.
Practical Application of STL Containers
One practical application of STL containers is distributing office templates and company images via SharePoint libraries. SharePoint uses a container-like structure to organize and manage these assets, demonstrating the wide-ranging applicability of STL containers’ concepts.
As we delve deeper into the intricacies of STL, we can see how it serves as a powerful tool in the developer’s arsenal. Its containers offer organized, efficient, and flexible data storage options, further enhancing the capabilities of your software applications.
Enhancing Content Delivery with the Standard Template Library
Regarding content delivery, particularly in the Office 365 Content Delivery Network (CDN), the Standard Template Library (STL) plays a pivotal role. STL’s flexible and efficient data storage solutions make it ideal for managing rich media assets such as images, audio, and video, ensuring smooth and efficient content delivery.
Role of STL in Content Delivery
Content delivery networks require efficient data handling and quick retrieval, and that’s where STL steps in. STL containers provide a standardized way to store and manipulate data, making them integral to content delivery strategies. In the context of Office 365 CDN, STL can be used to manage and deliver content assets swiftly and efficiently.
Managing Rich Media Assets with STL
STL’s versatile containers can store and manage a wide range of data types, including rich media assets. Whether it’s high-resolution images, audio files, or video clips, STL containers can handle them all. This ability makes STL ideal for managing rich media content in a CDN environment.
Benefits of Using STL for Content Delivery
The use of STL in content delivery brings numerous benefits. Its efficient data storage and retrieval mechanisms can significantly enhance the speed and performance of a CDN. Moreover, STL’s flexibility allows it to adapt to various data types and sizes, making it a robust solution for content delivery networks.
Overcoming Challenges in Using STL for Content Delivery
While STL offers numerous benefits, it also comes with its own set of challenges. For instance, managing large volumes of data can lead to memory management issues. However, these challenges can be effectively addressed with a deep understanding of STL and its components. Proper utilization of STL containers, algorithms, and iterators can help overcome these potential pitfalls and ensure smooth content delivery.
Mastering the Standard Template Library is crucial in content delivery. STL’s versatility and efficiency can significantly boost the performance of a CDN, making it an indispensable tool in the modern tech landscape.
Product Updates
Explore our latest updates and enhancements for an unmatched CDN experience.
Request a Demo
Free Developer Account
Unlock CacheFly’s unparalleled performance, security, and scalability by signing up for a free all-access developer account today.
CacheFly in the News
Learn About
Work at CacheFly
We’re positioned to scale and want to work with people who are excited about making the internet run faster and reach farther. Ready for your next big adventure?